How to Use jQuery Selectors?
jQuery has power of minimizing lines of code. jQuery would be the powerful tool for the DOM. jQuery is easy to select elements using selector engine, it’s easy syntax, which is based on the same syntax you would use to select elements in CSS by borrowing from CSS 1-3. jQuery selects or finds element on page by Element Id, it is jQuery option to getElementById. By using get element by id selector jQuery uses elements Id. Other selector jQuery uses is Get element by class. In this jQuery selector class name of the element is used to access the elements on page. jQuery also get element by attribute. Also by using hierarchy jQurey selects element on page. jQuery has filters that are also useful to get elements.We will see some samples of different types of selectors.
Element by ID
In the example below we will see a selection of an element by id.It is same as java scripts getElementById(). Using the id of element jQuery gets element on the page.It has every easy syntax.
<html>
<head>
<title>Get Element by ID</title>
<script src="http://ajax.microsoft.com/ajax/jquery/jquery-1.4.2.js"></script>
<script type="text/javascript">
//get element by it's ID
alert($("#message").text()); // Hello jQuery
</script>
</head>
<body>
<div id="message">Hello jQuery</div>
</body>
</html>
jQuery uses the native JavaScript function getElementById(), for id selectors. The fastest way to select an element is to reference it by its id selector.
It’s also important that your id should not contain the special characters like:
#;&,.+*~':"!^$[]()=>|/
The characters above if not properly escaped ay confuse the jQuery selector engine as it is a different type of selector. You can use escape character way like below to handle special characters.
$(“#some:id”) -> $(“#some\\:id”)
Get Elements by its class
In below example we will select all elements on the page that have the specified class name. jQuery uses the class of element and get the element from the page.
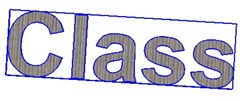
<html>
<head>
<title>Get Element by class selector</title>
<script src="http://ajax.microsoft.com/ajax/jquery/jquery-1.4.2.js"></script>
<script type="text/javascript">
//Get the element by it's classname
alert($(".message ").text()); // HellojQuery
</script>
</head>
<body>
<p id="hello">
<span class="message">Hello</span> <span class="message">jQuery</span>
</p>
</body>
</html>
In above example, selector matched both the span elements that have class= “message” and combined contents into one result ‘HellojQuery’.
jQuery will use the native JavaScript function GetElementsByClassName().
Get Elements by Attribute
Below example shows how to select elements that have an attribute with some specific value.
<html>
<head>
<title>Get Element by its attribute</title>
<script src="http://ajax.microsoft.com/ajax/jquery/jquery-1.4.2.js"></script>
<script type="text/javascript">
//get elements by its attribute
alert($("input[name= name]").val()); // johan
</script>
</head>
<body>
<form>
<p> Name: <input type="text" name="name" value="Johan" /> </p>
<p> Phone: <input type="text" name="phone" value="0000000" /> </p>
</form>
</body>
</html>
Here selector finds all matching ‘input’ elements then the element that have an attribute of ‘name’ and that also have the value ‘name’.
Get Elements by Hierarchy
Descendent Selector (“parent child”) – This will selects all descendent child elements of the parent including element which may a child, grandchild, great-grandchild, and so on, of that element.
Example:
<p><a href="http://google.com">Google</a></p>
<div>
<p> <a href="http://Yahoo.com">Yahoo!</a></p>
<p><a href="http://gmail.com">Gmail</a></p>
</div>
<ul>
<li><a href="http://jquerymobile.com">jQuery Mobile</a></li>
</ul>
<div>
<ul>
<li><a href="http://jquery.com">jQuery</a></li>
<li><a href="http://jqueryui.com">jQuery UI</a></li>
</ul>
</div>
When we write jQuery like:
$("div p");
It will give us below result:
<p> <a href="http://Yahoo.com">Yahoo!</a></p>
<p><a href="http://gmail.com">Gmail</a></p>
And if we write like below code
$("div ul");
then it’s result will be like:
<ul>
<li><a href="http://jquery.com">jQuery</a></li>
<li><a href="http://jqueryui.com">jQuery UI</a></li>
</ul>
Child Selector (“parent > child”) – This may be used when you need to selects all direct child elements of the parent.
Example:
<p><a href="http://google.com">Google</a></p>
<div>
<p> <a href="http://Yahoo.com">Yahoo!</a></p>
<p><a href="http://gmail.com">Gmail</a></p>
</div>
<ul>
<li><a href="http://jquerymobile.com">jQuery Mobile</a></li>
</ul>
<div>
<ul>
<li><a href="http://jquery.com">jQuery</a></li>
<li><a href="http://jqueryui.com">jQuery UI</a></li>
</ul>
</div>
When we write jQuery like:
$("p > a");
This will give us all the <a> tags:
<a href="http://google.com">Google</a>
<a href="http://Yahoo.com">Yahoo!</a></
<a href="http://gmail.com">Gmail</a>
And below code will be resulted as:
$("li > a");
Result of above code will be
<a href="http://jquerymobile.com">jQuery Mobile</a>
<a href="http://jquery.com">jQuery</a>
<a href="http://jqueryui.com">jQuery UI</a>
Get Elements by Filters
<!DOCTYPE html>
<html>
<head>
<title>Select Element by Selector Filter</title>
</head>
<body>
jQuery project sites:
<ul>
<li><a href="http://jquery.com">jQuery</a></li>
<li><a href="http://jqueryui.com">jQuery UI</a></li>
<li><a href="http://sizzlejs.com">Sizzle</a></li>
<li><a href="http://jquery.org">jQuery Project</a></li>
</ul>
<script src="http://ajax.microsoft.com/ajax/jquery/jquery-1.4.2.js"></script>
<script type="text/javascript">
//Select the element by attribute
alert($("a:first").attr("href")); // http://jquery.com
</script>
</body>
</html>
There are three types of filter selectors that you can used to get elements: numerical or positioned filters, CSS filters and Pseudo filters
Numerical or positioned filters:
:first< matched first Gives –>
:last - Gives last matched element
:first-child – Gives the first child element
:last-child - Gives the last child element
:only-child - Gives all element that have no siblings
:nth-child(n) - Gives the nth child element
:nth-child(even|odd) – Gives the matched even or odd children elements
:nth-child(xn+y) - Gives the nth matched children elements based on some formula.
:even - Gives even children elements
:odd - Gives odd children elements
:eq(n) - Gives nth position child element
:gt(n) - gives the children element those are greater than the nth position
:lt(n) - gives the children element those are less than the nth position
Traditional CSS filters:
:checked – It is basically used with checkboxes or radio buttons. It will return all checked elements.
:disabled – This will return all disabled elements
:enabled – This will give us all enabled elements
jQuery Pseudo filters:
:animated – Gives all elements with animations.
:button – Returns all button elements
:checkbox - Returns all checkbox elements
:contains(string) - Returns the elements those contain given string
Example: $(“div:contains(Google)”)
:file – Return all file input elements
:has(selector) –Returns elements that have at least one of the specified matched selector.
:header - Returns header elements.
:hidden – Returns all hidden elements
:image - Returns image input elements
:input - Returns form elements
:parent - Returns elements that have children, including text, which are not empty
:password - Returns elements of type password
:radio - Returns radio elements
:reset - Returns reset buttons
:selected - Returns option elements in selected state
:submit - Returns elements of type submit
:text - Returns elements of type text
:visible - Returns elements that are visible